Loading the payment page dynamically
Instead of redirecting your customers to the payment page, you can load the payment page dynamically in your webste.
Using an IFrame with the payment url poses a number of problems:
- You can't know the exact size of the payment page in order to avoid scrollbars
- You can't close the iFrame from within the iFrame due to cross-domain protection
To work around these limitations, you can display the payment page dynamically, using the DynamicJavascriptUrl returned from the createPaymentRequest method.
Integration
- When the customer selects the payment method (and optionally clicks pay), retrieve the DynamicJavascriptUrl from your server
-
Load the DynamicJavascriptUrl as a script
<script> function injectUrl(url) { $('body').append('<'+'script src="'+url+'"></'+'script>'); } </script>
Requirements
- The clients must have JavaScript enabled
- Your web server must use HTTPS to enable safe cross-origin communication using the window.postmessage() method. For more information, see https://developer.mozilla.org/en-US/docs/Web/API/Window/postMessage.
Dynamic Flow Behaviour Configuration
skipBodyOverflow
placePaymentWindow
Support Dynamic Flow
The dynamic flow will allow your website to react upon changes in the status of the payment, so you can take decisions as to what to render to the customer, where to redirect to, and in which conditions.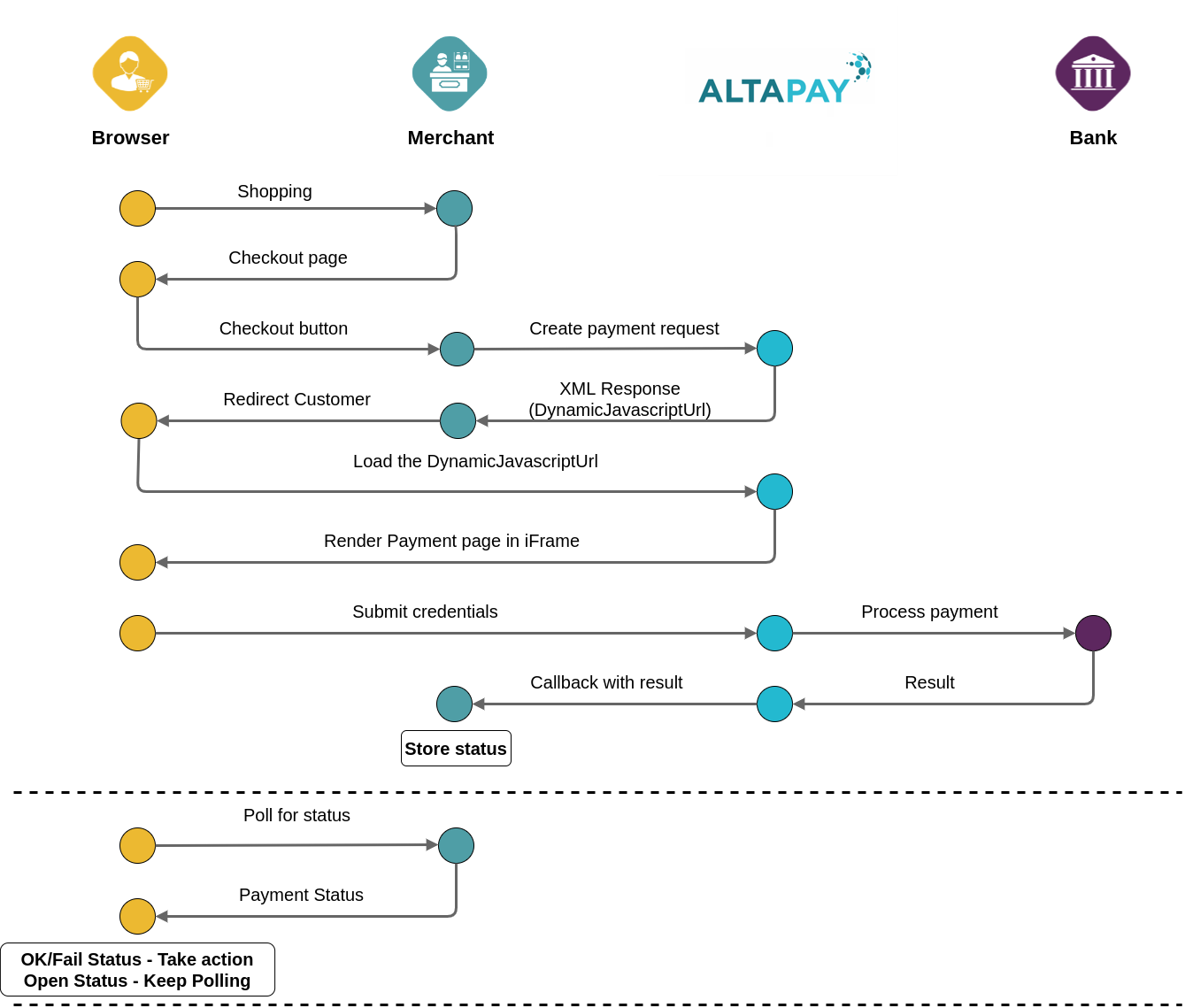
Merchant customized solution
This integration requires a bit more work on your end, but gives you full flexibility about how the payment flow will react and behave.- When you load the DynamicJavascriptUrl, keep polling your server for status
- Store in your server the status of the payment upon callback_ok,callback_fail, or callback_notification
- When the status is final, the polling mechanism should automatically close the window, and redirect the customer to your final page (or load it dynamically)
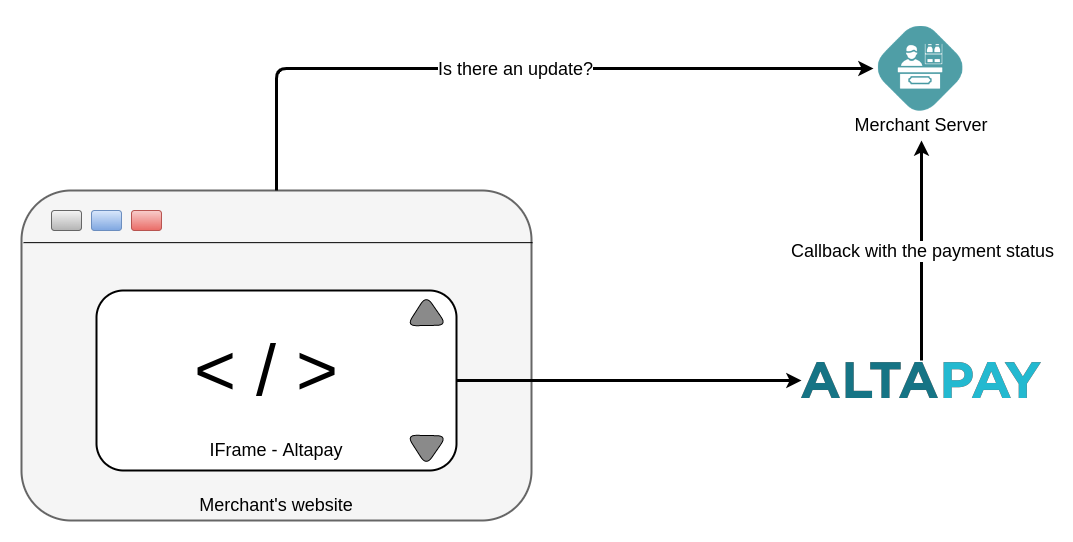
AltaPay native solution
This integration allows for basic operations like closing the payment window, redirecting the customer, or scheduling a specific payment event, all without communication between your website and your backend.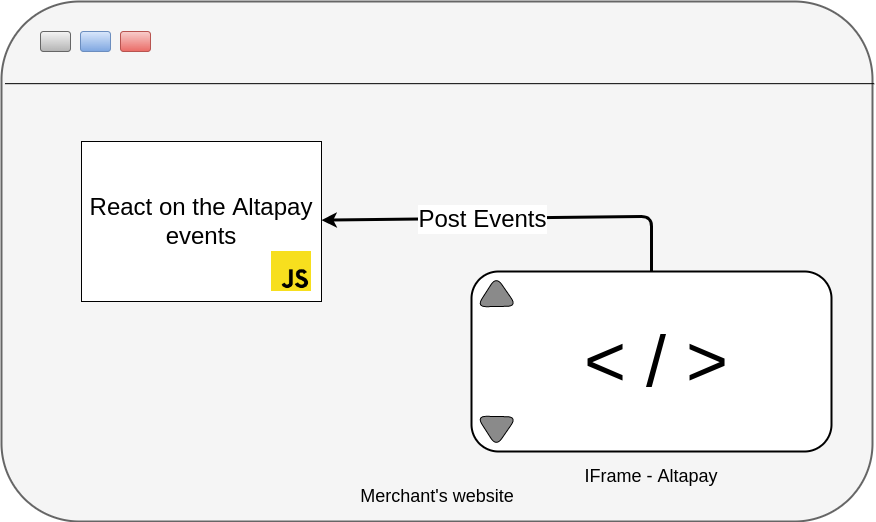
The iFrame content will automatically post the following events:
- payment_completed - when the payment ends successfully
- payment_failed - when the payment is failure
Both events provide the following parameters:
Parameter | Description | Type | ||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
status |
The status of the transactions:
|
String | ||||||||||||||||
currency | This is the payment currency. It is specified in an ISO-4217 format, either using the 3-digit numeric code, or the 3-letter character code. For more information about ISO-4217 currency codes, see https://en.wikipedia.org/wiki/ISO_4217. | [0-9]{3} or [A-Z]{3} | ||||||||||||||||
amount | This is the payment amount. It uses a dot as the decimal separator, and the amount can have maximum 2 decimals. | float | ||||||||||||||||
transaction_info | This is a one-dimensional associative array, where you put any value that you would like to associate with the payment in the call to createPaymentRequest. | float | ||||||||||||||||
error_message | A fallback error message that is safe to display to your customers. You are recommended to always display your own, custom error message, providing your customer with more details about the error, and only use this error message as a fallback mechanism. You must include the message for Klarna payments , or if the cardholder_message_must_be_shown return parameter is true. | String | ||||||||||||||||
merchant_error_message | An error message for the merchant's eyes only, but provides more detail as to what went wrong (This is only sent to the FAIL-callback page). | String | ||||||||||||||||
cardholder_message_must_be_shown | An indicator on whether the card holder error message must be shown to your customer. | boolean | ||||||||||||||||
shop_orderid |
This is the internal ID of the order in your webshop. |
[a-zA-Z0-9-]{1,100} | ||||||||||||||||
payment_id |
The ID of the transaction. This should be stored if you are going to capture/cancel the order directly from your system, for example, for capturing reservations using the captureReservation method. |
[0-9a-f]{1-36} | ||||||||||||||||
transaction_id |
The ID of the payment. |
[0-9a-f]{36} | ||||||||||||||||
type |
The authorisation type of the payment, for example, "payment"/"paymentAndCapture"/"subscription" |
String | ||||||||||||||||
payment_status |
The status of the transaction, depending on the payment method, for example, preauth,recurring_confirmed, captured, etc. |
String | ||||||||||||||||
masked_credit_card |
The masked primary account number of the credit card, which contains the maximum number of digits allowed to share with a non-PCI compliant merchant. |
String | ||||||||||||||||
blacklist_token |
A unique token you can use for blacklisting a credit card. This token can be used to build up a black list of credit cards. The token is only returned if this is a credit card payment. |
[0-9a-f]{41} | ||||||||||||||||
credit_card_token |
This is the unique credit card token value that identifies a credit card within the AltaPay Payment Gateway. This token can be used later with the Merchant API or with creditcard token ordering so that the customer does not need to enter credit card details in their next order. The token is only returned for credit card payments. |
[0-9a-f]{41} | ||||||||||||||||
nature |
The payment method:
|
String | ||||||||||||||||
require_capture |
Indicates whether a transaction was just reserved, and subsequently needs to be captured. For example, if a credit card payment has the authorisation type "payment", this value is true, but if the authorisation type is paymentAndCapture, this value is false, because the transaction has already been captured. |
boolean | ||||||||||||||||
fraud_risk_score |
This is the probability that the transaction is a fraud attempt, and should be read in context of the FraudExplanation and the FraudRecommendation. For example a fraud risk score of 13.37 means there is a 13.37% chance of this order being fraud. Only MaxMind utilizes the fraud score. For ReD, the value is always -1. |
float | ||||||||||||||||
fraud_explanation | Contains a detailed explanation of the fraud risk evaluation. Only relevant for MaxMind. For ReD it contains the value of the recommendation. | string | ||||||||||||||||
fraud_recommendation |
The recommendation from the fraud detection service provider. For example:
Unknown is also returned for declined transaction.
|
String | ||||||||||||||||
callback_result |
Based on the step value, the native integration will react automatically or, your will have to react on the events. Possible values:
In all the cases,your website can listen to the payment_completed/payment_failed events and can react in a custom way. |
String |
The AltaPay native solution will automatically react on the events as follows:
-
callback_ok/callback_fail not defined for the transaction
- AltaPay default success/fail response page is shown inside iFrame.
-
callback_ok/callback_fail defined for the transaction
-
callback_ok/callback_fail responds with HTTP 3xx redirection response - AltaPay native solution will automatically close the iFrame and will do the redirection.
If you what the callback_ok/callback_fail redirect response to finalize inside the iFrame, you should specify this in a parameter called handleRedirectInsideIFrame with value true passed in the /eCommerce/API/embeddedPaymentWindow GET call.
In this case, the payment_completed/payment_failed events will not be sent.
https://testgateway.altapaysecure.com/eCommerce/API/embeddedPaymentWindow?pid=abcd123 is the DynamicJavascriptUrl retrieved in the /merchant/API/createPaymentRequest response that is going to load embedded payment window. You'll have to add the handleRedirectInsideIFrame parameter to ot so that the URL loaded in the script will be: https://testgateway.altapaysecure.com/eCommerce/API/embeddedPaymentWindow?pid=abcd123&handleRedirectInsideIFrame=true
- callback_ok/callback_fail responds with HTTP 2xx success response - AltaPay native solution will display the content of the response inside the iFrame. Your website can listen to the events and react.
- callback_ok/callback_fail with HTTP 2xx success response with having 'OKAY' string as content - AltaPay native solution will automatically close the iFrame. Your website can listen to the events and react.
-
callback_ok/callback_fail responds with HTTP 3xx redirection response - AltaPay native solution will automatically close the iFrame and will do the redirection.
Your website javascript can listen to the payment_completed/payment_failed events in all the above described scenarios (except the case when handleRedirectInsideIFrame=true) and use the parameters to define a custom behavior. This offers you flexibility to define the customer experience without interaction with your backend.
Styling